- 깃허브에 커밋해서 문제풀이 블로그 포스팅은 잘 안했지만 재미로 쉬운거만 계속 풀고 있음
- 요새는 테스트케이스를 미리 추가해놓고 푸는 방식으로 하는 중
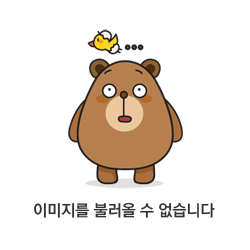
// Process
// 1. Input 2 string arrays
// 2. 한개 배열씩 반복한다.
// 2.1. 중복안되는 것들의 배열을 각각 만든다.
// 3. 중복안되는 것들의 배열 2개에서 서로 중복되는 개수를 센다.
// 4. 개수 반환한다.
public int countWords(String[] words1, String[] words2) {
int result = 0;
Set<String> repeated = new HashSet<>();
Set<String> set1 = new HashSet<>();
Set<String> set2 = new HashSet<>();
// 2.
for (String word : words1) {
if (repeated.contains(word)) {
continue;
}
if (set1.contains(word)) {
set1.remove(word);
repeated.add(word);
continue;
}
set1.add(word);
}
for (String word : words2) {
if (repeated.contains(word)) {
continue;
}
if (set2.contains(word)) {
set2.remove(word);
repeated.add(word);
continue;
}
set2.add(word);
}
// 3.
for (String string : set1) {
if (set2.contains(string)) {
++result;
}
}
return result;
}
테스트코드는 assertj 라이브러리를 씀
import static org.assertj.core.api.Assertions.assertThat;
import org.junit.jupiter.api.Test;
public class LeetCode_2085_CountCommonWordsWithOneOccurrenceTest {
@Test
public void 두개의_배열에서_각각_1회씩만_나오면서_겹치는_숫자의_개수() {
LeetCode_2085_CountCommonWordsWithOneOccurrence test = new LeetCode_2085_CountCommonWordsWithOneOccurrence();
assertThat(test.countWords(new String[] {"leetcode", "is", "amazing", "as", "is"}, new String[] {"amazing", "leetcode", "is"})).isEqualTo(2);
assertThat(test.countWords(new String[] {"b","bb","bb"}, new String[] {"a","aa","aaa"})).isEqualTo(0);
assertThat(test.countWords(new String[] {"a", "ab"}, new String[] {"a","a", "a", "ab"})).isEqualTo(1);
}
}
'Programming' 카테고리의 다른 글
Oracle to MySQL 변환 시 고려사항 (RDBMS) (0) | 2021.12.15 |
---|---|
[LeetCode] 1742. Maximum Number of Balls in a Box (TDD, 코테, 릿코드, tech interview) (0) | 2021.12.11 |
Gradle 의존 키워드의 차이점 (compile vs implementation + api, Gradle dependency configurations diff) (0) | 2021.11.28 |
RPC, 근데 이제 Json을 곁들인.. (Json-RPC) (0) | 2021.11.17 |
개발자의 글쓰기 - 책정리 (0) | 2021.10.26 |